ONLINE PAYMENT USING PAYPAL IPN WITH CODEIGNITER.
(SESSION CAN BE USED IF DATABASE IS NOT INVOLVED IN THE PAYMENT PROCESS )Introducing Instant Payment Notification (IPN)
Instant Payment Notification (IPN) is a message service that automatically notifies merchants of events related to PayPal transactions. Merchants can use it to automate back-office and administrative functions, including automatically fulfilling orders and providing customers with order status.
Why use Instant Payment Notification
Instant Payment Notification (IPN) notifies merchants almost instantly about transaction events, such as:
How it works
Merchants create an Instant Payment Notification(IPN) listener page on their website and then specify the URL of the listener page in their PayPal account profile. PayPal then sends notifications of all transaction-related events to that URL. When customers pay for goods or services, PayPal sends a secure FORM POST containing payment information (IPN messages) to the URL. The Instant Payment Notification(IPN )listener detects and processes Instant Payment Notification(IPN) messages using the merchant backend processes. The Instant Payment Notification (IPN) listener page contains a custom script or program that waits for the messages, validates them with PayPal, and then passes them to various backend applications for processing.
Referral to this link for paypal (IPN) Instant Payment Notification
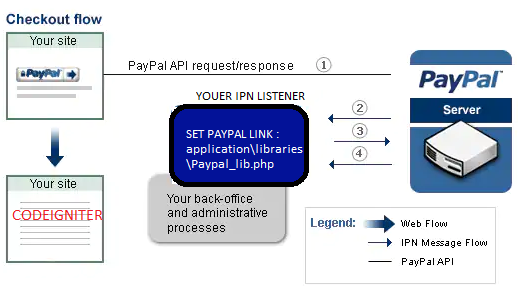
I will share with you integration of PayPal gateway payment without database in codeigniter. In this blog you will learn how to integrate Paypal payment gateway without database in Codeigniter. This blog will give you full example of the PayPal payment gateway integration in the Codeigniter.
In this example, I will show you easy and simple way to integrate paypal payment gateway in codeigniter . I will show you step by step to integrate paypal payment gateway in codeigniter as follow.
Step 1: Set link in paypal after Instant Payment Notification (IPN ) file is ready
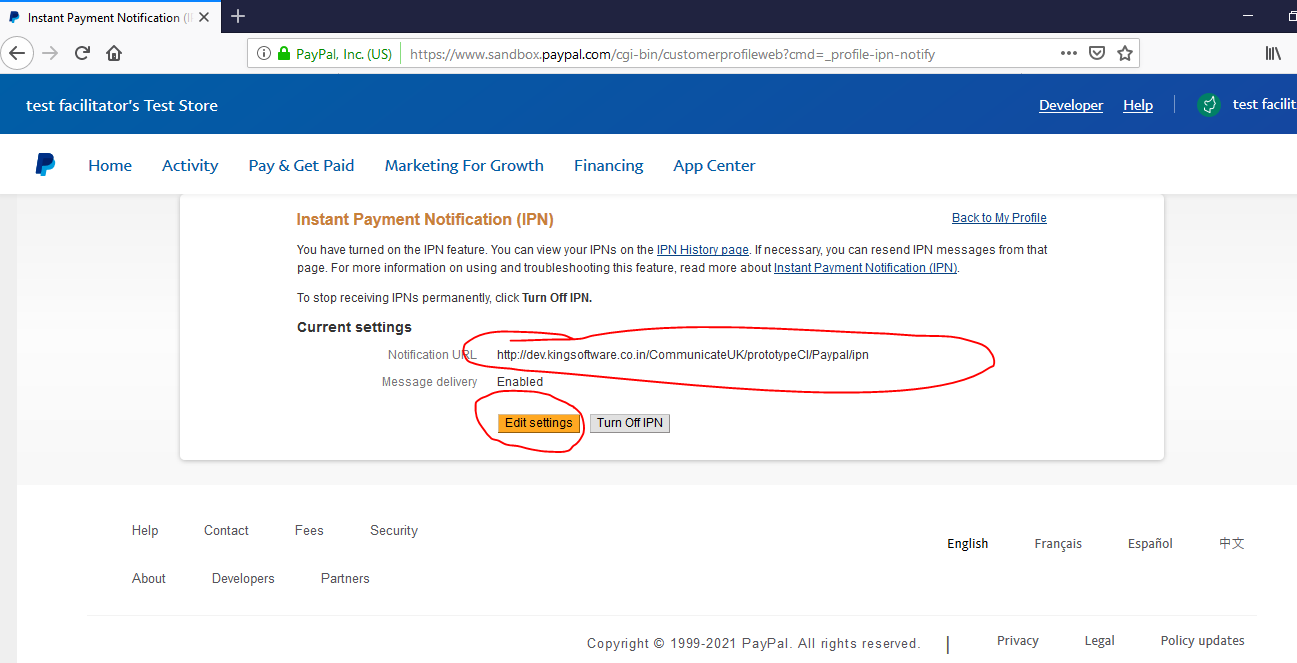
Step 2: Create a Payment Form payment_form.php.
view/payment_form.php
<form action="<?php echo base_url('Payment/paypal_online_payment_form_Validation');?>" class="form-validation contact-form" autocomplete="off" method="post" onsubmit="return validate('Payment_Form');" style="margin-top: 0%" id="contact" >
<lablel>Name </lablel>
<input type="text" name="fname" id="fname" placeholder="Your name" >
<lablel>Email </lablel>
<input type="text" name="email" id='email' placeholder="Email address" >
<lable>Amount To Paid</lable>
< input type="text" name="amount" id='amount' placeholder="Amount"
<button class="theme-btn btn-style-one" type="submit" name="submit-form"><span class="txt">Submit now<span></button>
</form>
Step 3: : Take data from form to payment_confirmation.php page and store in to a Session. And Process data for Paypal.
view/payment_confirmation.php
<form id="Payment_Form" action=" <?php echo base_url('Payment/pay');?>" method="post" >
<input type="hidden" name="fname" value="<php echo $fname;?>"
<input type="hidden" name="email" value="<php echo $email ; ?>">
< input type="hidden" name="amount" value="<php echo $amount; ?>">
<input type="image" class="imgbtn" name="submit" src="https://www.paypal.com/en_GB/i/btn/btn_paynowCC_LG.gif" alt="Check out with PayPal" />
</form>
Step 4: We’ll create two controllers in codeigniter, Payment and Paypal.
controller/Payment
-Data arrives from the paymet_form and Payment controller pay() method stores the session data and passes the session data to the PayPal controller store data to the success method.
-pay() method load the paypal data though session
-This line add after Add fields to paypal form
public function pay() {
//Load paypal form
$session_data = array('fname' => $name, 'email' => $_POST['email']);
$this->session->set_userdata($session_data);
$this->paypal_lib->paypal_auto_form();
}
Step 5: controller in Codeigniter : Paypal
-This controller has four methods: success(), ipn(), PaymentSuccess(), sendEmailforPaymentdetails().
function success(){
// Get the transaction data
$paypalInfo = $this->input->get();
$details=explode(',',$paypalInfo['item_name']);
$data['fname']=$details[0];
$data['email'] = $details[1];
$data['payment_amount'] = $paypalInfo["amt"];
// Pass the transaction data to view
$data['page_name'] = "success";
$this->session->set_userdata('paypal_data', $data);
// redirect data to paymentsuccess
redirect('Paypal/paymentSuccess');
}
-Retrieve the transaction data from the query string of the URL.
-Pass the payment data to the view and load the view file.
-In this success(), method remove the email shoot process and add this process IPN method in the last section so email shout to IPN and this process is sent to email to customer and manager
-success() method redirect data to paymentsuccess() method
function paymentSuccess(){
//store data through session
$data = $this->session->userdata('paypal_data');
$this->load->view('layout/main_layout',$data);
}
-paymentSuccess() method store the data through the session in the success() method
function sendEmailforPaymentdetails($data)){
if(!empty($data)) {
$bodydata['fname']=$name=$data['fname'];
$bodydata['email']=$email = $data['email'];
$bodydata['payment_amount']=$data['payment_amount'];
$subject = "kingsoftware Payment Confirmation";
$from="test@kingsoftware.co.in";
$to=$email;
$cc="";
$bcc="";
$attach="";
$body=$this->load->view('email_template/payment_receipt',$bodydata,true);
$reply=$this->Prototype_email_m->sendMail($from,$name,$to,$cc,$subject,$body,$attach,$bcc);
return $reply;
}
}
-sendEmailforPaymentdetails() method is used to shoot emails to customers and the manager
function ipn(){
// Retrieve transaction data from PayPal IPN POST
$paypalInfo = $this->input->post();
$details=explode(',',$paypalInfo['item_name']);
$data['fname']=$details[0];
$data['email'] = $details[1];
$data['phone'] = $details[2];
$data['payment_amount'] = $paypalInfo["mc_gross"];
$paypalURL = $this->paypal_lib->paypal_url;
$result = $this->paypal_lib->curlPost($paypalURL,$paypalInfo);
//check whether the payment is verified
if(preg_match("/VERIFIED/i",$result)){
//if verified mail is shoot
if( $this->sendEmailforPaymentdetails($data);
}
}
-This method is called by the PayPal IPN (Instant Payment Notification).
Paypal retrieve the all transaction data from PayPal IPN (Instant Payment Notification)
In the ipn() function of Paypal controller, each transaction is verified and shoot the mail to customer and the manager. This paypal/ipn URL is called by the PayPal IPN and it must be enabled in PayPal.